Here’s a toy program I wrote today in Python 3.6, to try out the asyncio.Queue
object. One coroutine pushes an item to the queue every second, and two more fetch them from the queue and “process” them. Over time, the processors are too slow and the queue builds up.
Of special note is the fact that the two processors never get the same item. That’s pretty neat when you think about it.
And it’s all a single thread! If these processors were based on I/O, you could run a lot more than two in parallel.
1 |
|
Sample output:
1 |
|
As always, a huge thanks to Dash for making it easy to explore random libraries. And to a lesser extent, Alfred for providing lookups almost at the speed of thought.
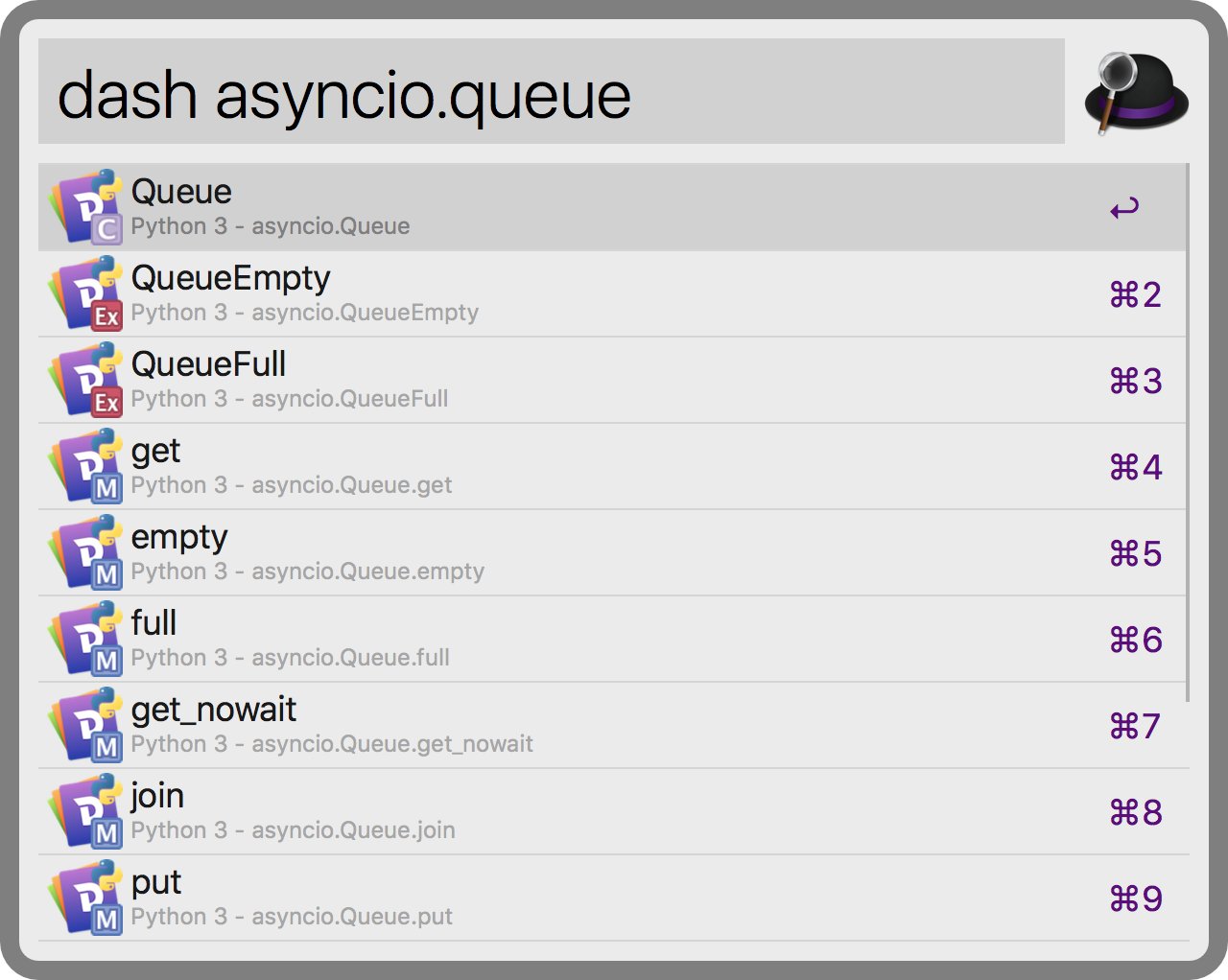
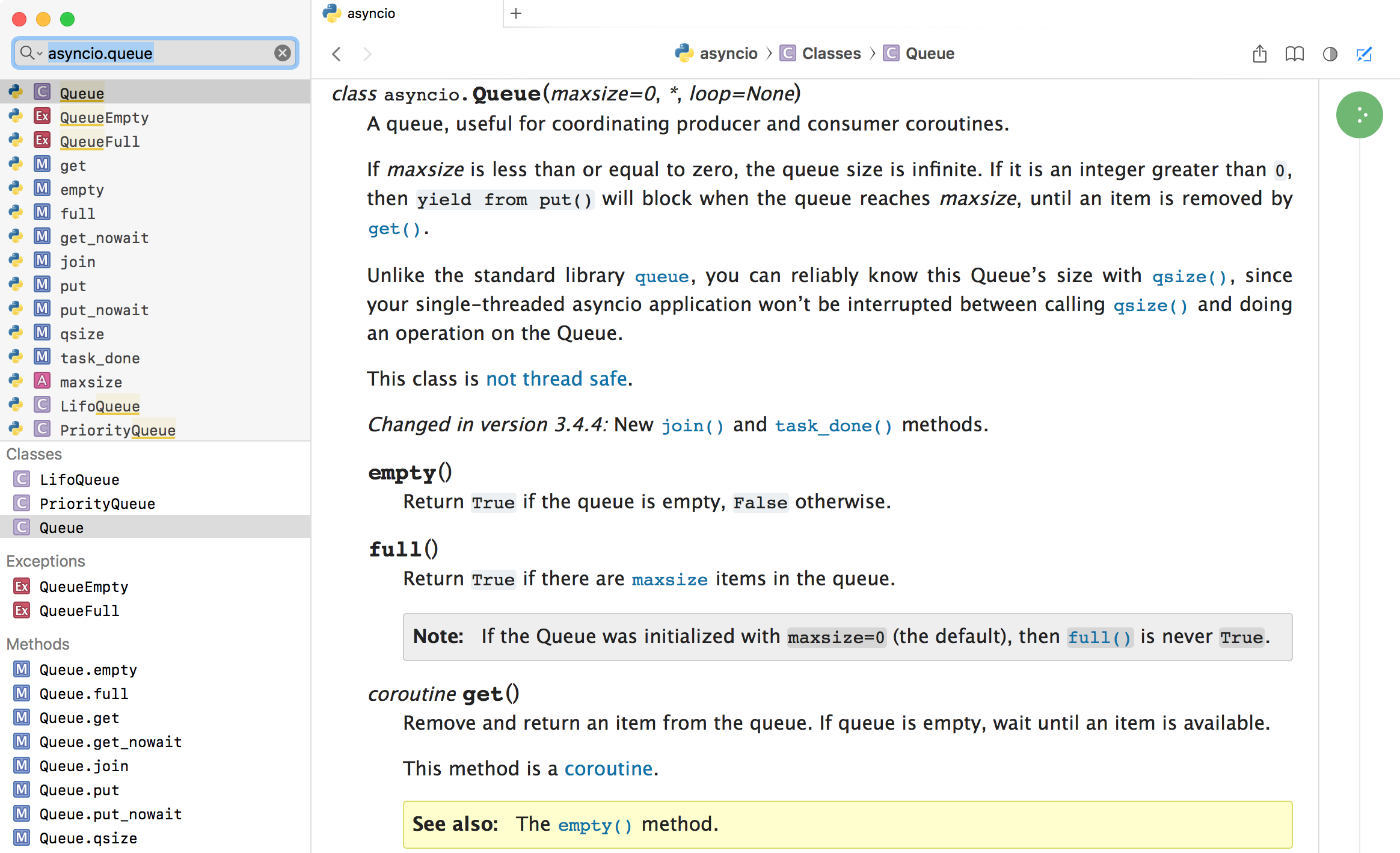